Example usage of tigerFactory() executions
When you create a new frontend application using npx @gooddata/create-gooddata-react-app@latest my-app
, the CLI walks you through some configuration steps where you fill in your hostname and pick a desired JavaScript flavor. The boilerplate then contains all necessary logic so that you can authenticate and start building your custom frontend application on top of the GoodData platform. But what if you don’t want to use the @gooddata/create-gooddata-react-app CLI, maybe you don’t even want to use React.
This article describes how to instantiate the GoodData backend in a framework agnostic environment. To get a head start on webpack configuration you can use popular webpack Boilerplate by Tania Rascia:
git clone git@github.com:taniarascia/webpack-boilerplate.git
cd webpack-boilerplate
npm i
npm start
After running the above command you should see the homepage of the webpack boilerplate that should look something like this:
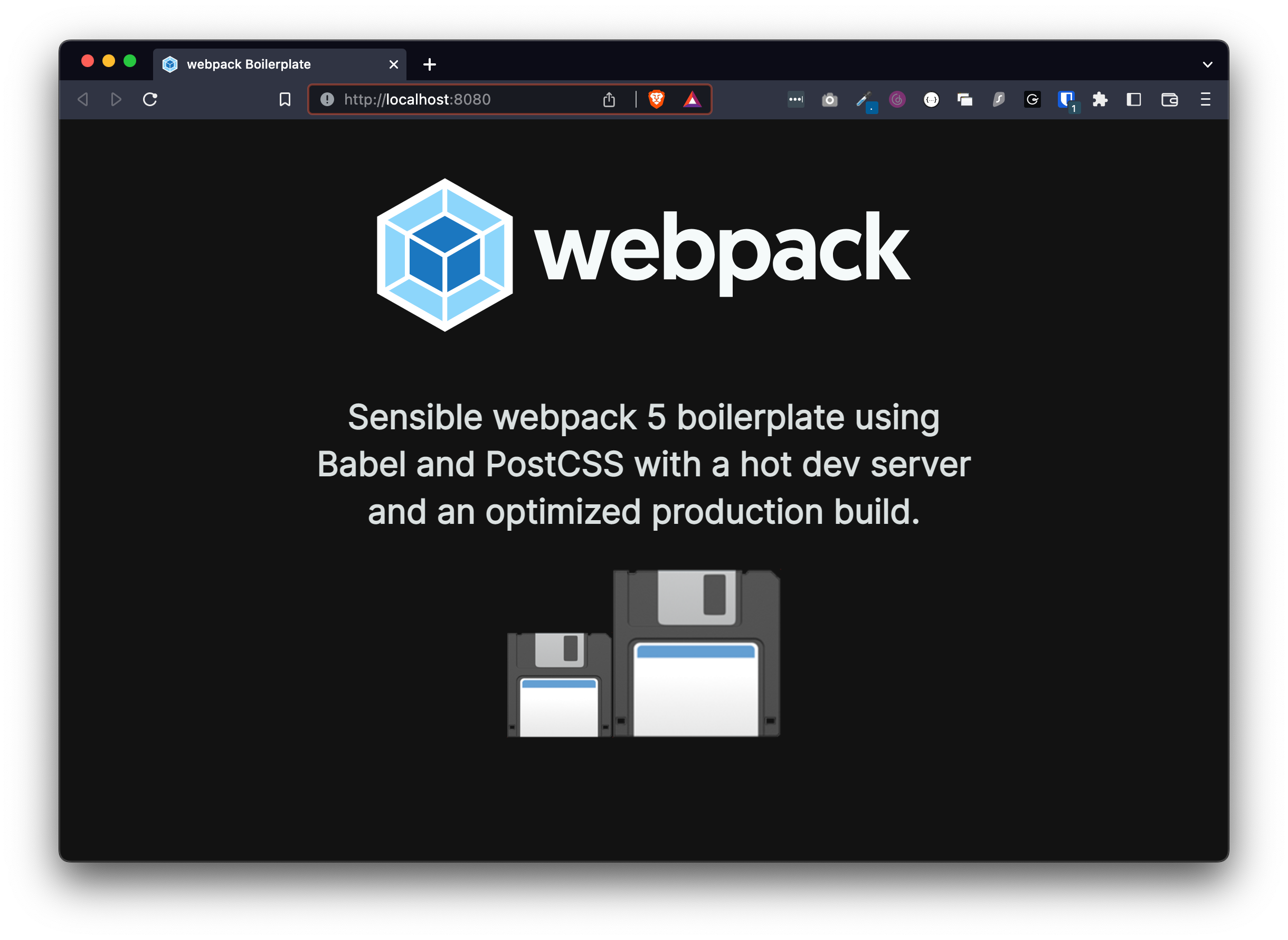
First, let’s add some GoodData dependencies:
npm install @gooddata/sdk-backend-tiger
npm install @gooddata/sdk-model
You can double check your package.json
file that the dependencies were successfully added.
Make sure that all of the GoodData dependencies have the exact same version!
Next, navigate to src/index.js
and add the following imports to the top of the file:
import {
idRef,
newMeasure,
newAttribute,
newTwoDimensional,
MeasureGroupIdentifier
} from "@gooddata/sdk-model";
import tigerFactory, { TigerTokenAuthProvider } from "@gooddata/sdk-backend-tiger";
Now, paste the following code to the bottom of the src/index.js
file:
const backend = tigerFactory({
hostname: "https://jirizajic.demo.cloud.gooddata.com/"
}).withAuthentication(
new TigerTokenAuthProvider('getApiToken') // https://use.gd/getApiToken
);
const metric = newMeasure(idRef("average_price_of_products", "measure"));
const viewBy = newAttribute("product_brand");
const stackBy = newAttribute("product_category");
const filters = [];
Inside React framework
If you’re using React you can utilize the GoodData.UI Components like this:
<div style={{ height: 400 }}>
<ColumnChart
backend={backend}
workspace="yourWorkspaceId"
measures={[metric]}
viewBy={viewBy}
stackBy={stackBy}
filters={filters}
/>
</div>
Framework-agnostic approach
If you’re not using React you can do the following to get the raw data via JavaScript Promises:
const execution = backend
.workspace('yourWorkspaceId')
.execution()
.forItems([metric, viewBy, stackBy], filters)
.withDimensions(
...newTwoDimensional(
[viewBy],
[MeasureGroupIdentifier, stackBy]
)
);
execution.execute().then(result =>
result.readAll().then(data => console.log(data)));
Assuming everything goes according to the plan, you should see the dataview logged into your browser console similar to the screenshot below:
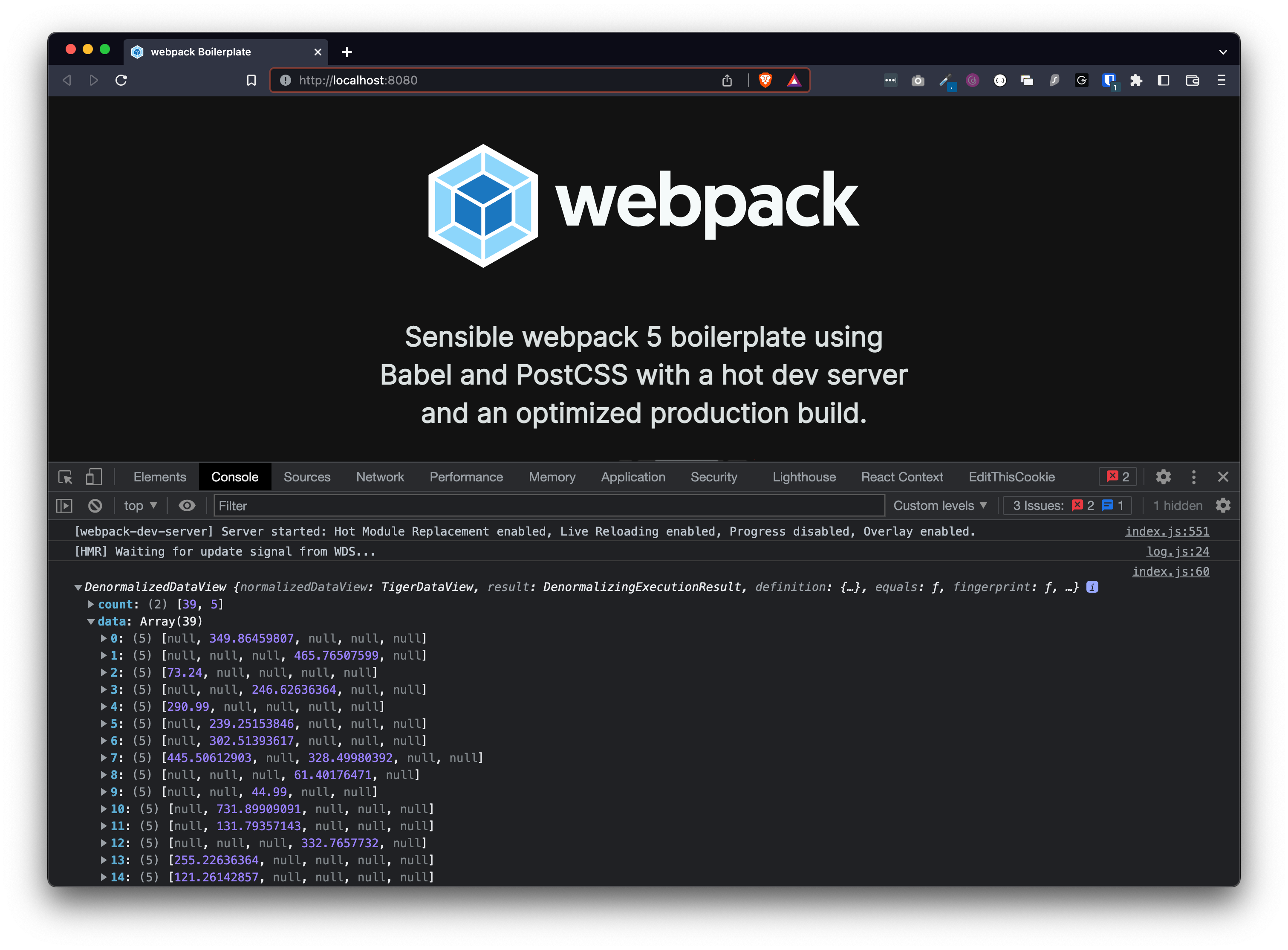
Troubleshooting
If you’re running into a CORS issue similar to the example below, see how to enable CORS for your organization.
Access to XMLHttpRequest at 'https://jirizajic.demo.cloud.gooddata.com/api/v1/actions/workspaces/ecommerce-parent/execution/afm/execute' from origin 'http://localhost:8080' has been blocked by CORS policy: Response to preflight request doesn't pass access control check: No 'Access-Control-Allow-Origin' header is present on the requested resource.
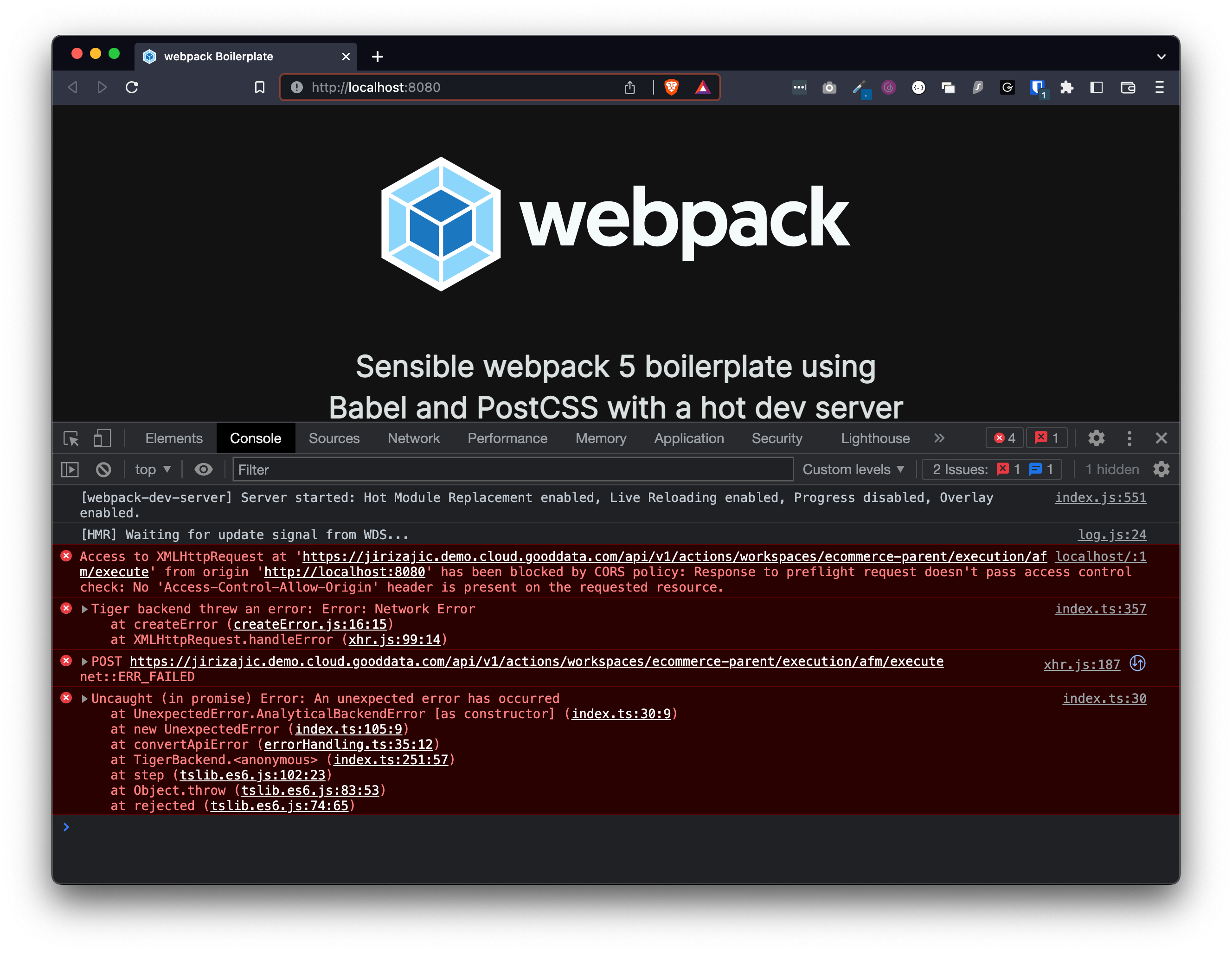
Â